设计动机
- 在软件系统中,经常有这样一些特殊的类,必须保证它们在系统中只存在一个实例,才能确保它们的逻辑正确性、以及良好的效率。
- 绕过常规的构造器,提供一种机制来保证一个类只有一个实例
- 这是类设计者的责任,而不是使用者的责任。
定义
保证一个类仅有一个实例,并提供一个该实例的全局访问点。
结构
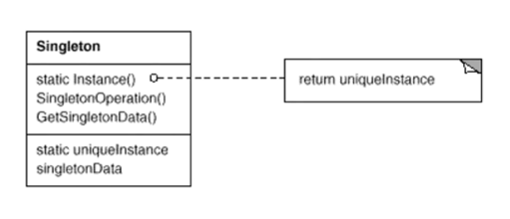
例子
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64
| class Singleton{ private: Singleton(){} Singleton(const Singleton&) = delete; Singleton& operator=(const Singleton&) = delete; public: static Singleton* getInstance(); static Singleton* m_instance; }
Singleton* Singleton::m_instance = nullptr;
Singleton* Singleton::getInstance(){ if(m_instance == nullptr){ m_instance = new Singleton(); } return m_instance; }
Singleton* Singleton::getInstance(){ Lock lock; if(m_instance == nullptr){ m_instance = new Singleton(); } return m_instance; }
Singleton* Singleton::getInstance(){ if(m_instance == nullptr){ Lock lock; if(m_instance == nullptr){ m_instance = new Singleton(); } } return m_instance; }
std::atomic<Singleton*> Singleton::m_instance; std::mutex Singleton::m_mutex;
Singleton* Singleton::getInstance() { Singleton* tmp = m_instance.load(std::memory_order_relaxed); std::atomic_thread_fence(std::memory_order_acquire); if (tmp == nullptr) { std::lock_guard<std::mutex> lock(m_mutex); tmp = m_instance.load(std::memory_order_relaxed); if (tmp == nullptr) { tmp = new Singleton(); std::atomic_thread_fence(std::memory_order_release); m_instance.store(tmp, std::memory_order_relaxed); } } return tmp; }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| class Singleton{ private: Singleton(){} Singleton(const Singleton&) = delete; Singleton& operator(const Singleton&) = delete; public: static Singleton* getInstance(){ static Singleton m_instance; return &m_instance; } }
|
- 单线程下,正确。
- C++11及以后的版本(如C++14)的多线程下,正确。
- C++11之前的多线程下,不一定正确。原因在于在C++11之前的标准中并没有规定local static变量的内存模型。于是乎它就是不是线程安全的了。但是在C++11却是线程安全的,这是因为新的C++标准规定了当一个线程正在初始化一个变量的时候,其他线程必须得等到该初始化完成以后才能访问它。
上述使用的内存序:
memory_order_relaxed:松散内存序,只用来保证对原子对象的操作是原子的
memory_order_acquire:获得操作,在读取某原子对象时,当前线程的任何后面的读写操作都不允许重排到这个操作的前面去,并且其他线程在对同一个原子对象释放之前的所有内存写入都在当前线程可见
memory_order_release:释放操作,在写入某原子对象时,当前线程的任何前面的读写操作都不允许重排到这个操作的后面去,并且当前线程的所有内存写入都在对同一个原子对象进行获取的其他线程可见
总结
- Singleton模式中的实例构造器可以设置为protected以允许子类派生。
- Singleton模式一般不要支持拷贝构造函数和Clone接口,因为这有可能导致多个对象实例,与Singleton模式的初衷违背。
- 如何实现多线程环境下安全的Singleton?注意对双检查锁的正确实现。
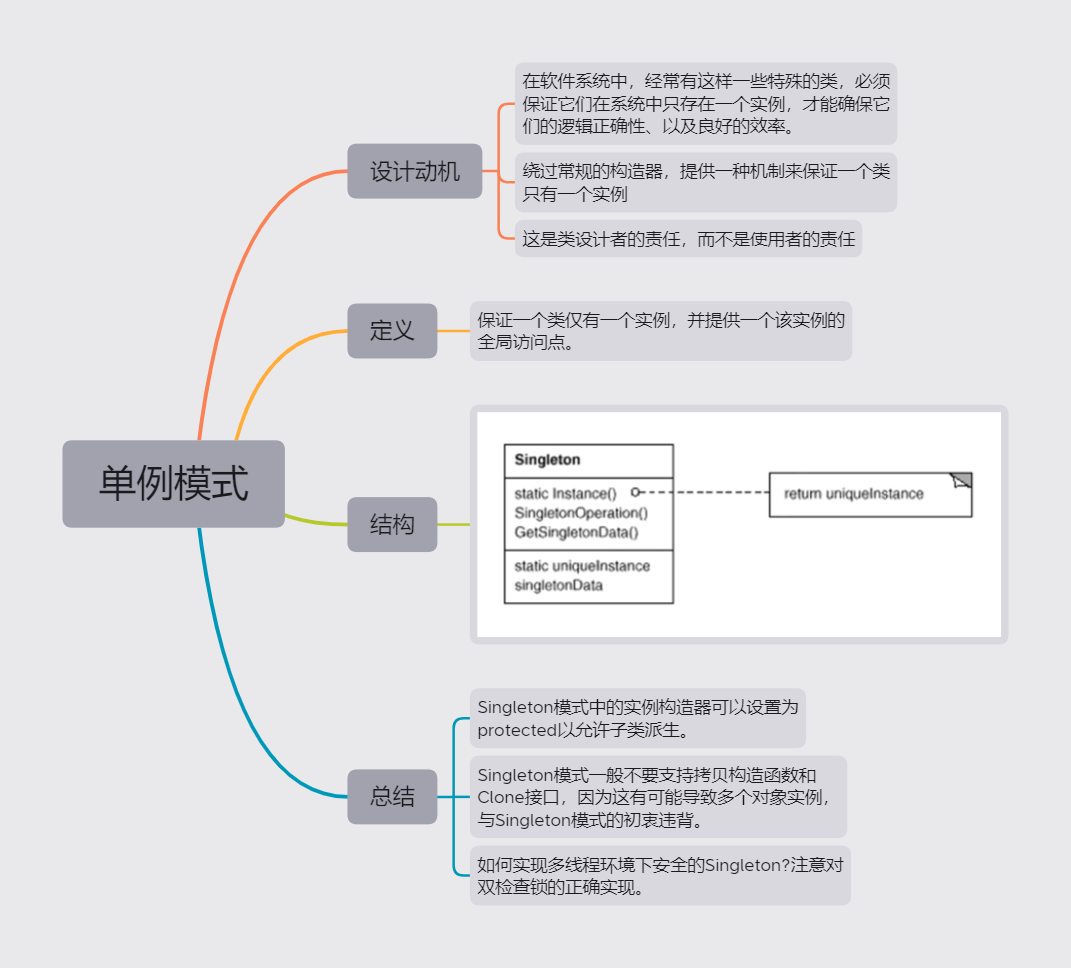